TAGS: #javascript
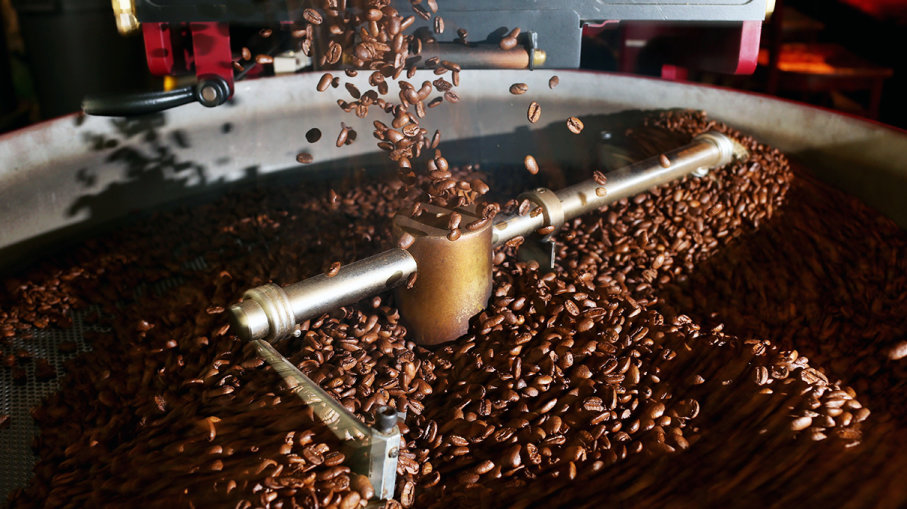
Once an HTML canvas tag has been added to your webpage you will need to define its size and context, which can only be done in JavaScript. Resizing the canvas is important for obvious reasons: It has to be big enough to provide sufficient space for your game or animation that you want to run inside the canvas. A less obvious characteristic of the canvas is the context. In this article, I will explain a bit more about the context, why we need it and finally how you can set the canvas size and context in JavaScript for a 2D browser game.
The Rendering Context for Your HTML5 Browser Game
If you search for definitions online of rendering context you will get very technical explanations. Moreover, it usually depends on the API that you are working with. Since we are working with the canvas we are using the HTML5 Canvas API for JavaScript. In this sense, the rendering context contains all the information to render a renderable object. It also includes paths to the object and any other request parameters. I think it’s best if I give you a simple example in JavaScript: context.fillRect(). If we have defined the context as 2D, we can then use the aforementioned method on the context to draw a rectangle on the canvas. This is only possible if we have set the context. For a complete list of all methods you should consult the official specifications page from Mozilla.
How to Set the Canvas Size and Context in JavaScript
Now that we know a bit more about the rendering context and understand why we need it, we can finally write the code. Writing the code is always the easiest part once you understand the big picture. Let’s start by setting the width and height of the canvas. For a regular game screen that fits into most desktop browsers I use a width of 800 pixels and a height of 480 pixels. Later on we can add an options menu to change the size but when developing this is my favorite size. To do this, you first need to save the HTML canvas element in a JavaScript variable that I suggest you name canvas, too. Now you can set the width as follows: canvas.width = 800. The same way you can set the height to 480. If you refresh your browser window you will now see the canvas has changed the size. Setting the context to 2D requires only the following single line of code: ctx = canvas.setContext(“2D”). As you noticed, I use the variable ctx instead of context. I do this because I prefer shorter variable names.